About Lesson
Mocking is utilized to supply mock data. For instance, if test “A” depends on functionality from tests “B” and “C”, we assume that “B” and “C” operate correctly. By using mock data for “B” and “C”, we can focus solely on writing the code for test “A”.
In the example below, we have a controller calling the getProduct method from a service object. If an issue arises in the service file, we cannot attribute it to the controller’s getProduct method. Here, we anticipate data from a third party, such as the service. To prevent this potential for flaky tests, we consistently return a mock product object, assuming it functions correctly. We then conduct tests based on this mock object and assert to ensure the required functionality
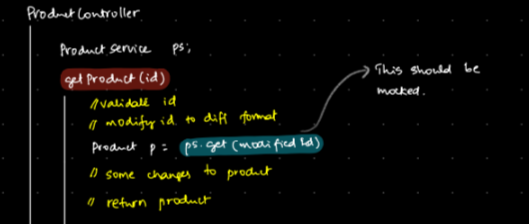
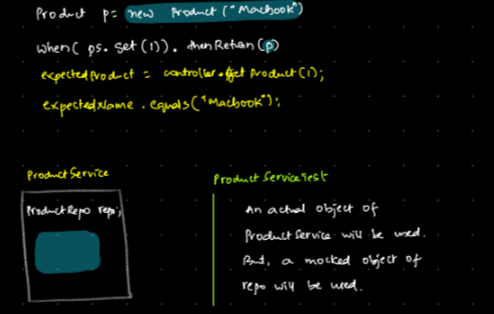
Double -> It’s like a body double in movies, where a stunt men replaces the actual actor. In the realm of testing, we have three types of doubles:
1. Mock 2. Stub 3. Fake
Mock -> One limitation of mocks is that they always produce the same result. For example, in a service test, if we create a mock repository object and call addProduct to add five products, and then call getProductCount, it will always return 5. If we subsequently add another product and expect 6, the mock will still return 5 because it lacks the capability to reflect changes in real data.
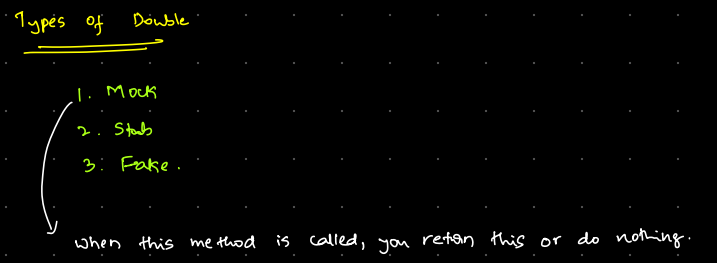
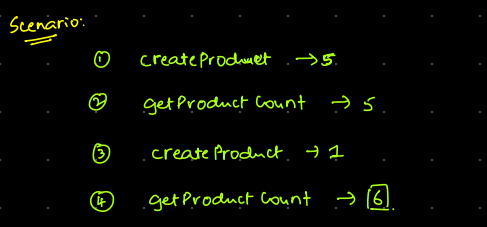
Stub -> A stub in unit testing is a simplified implementation of a component or module that provides predetermined responses to method calls or queries. It is used to simulate the behavior of real components or dependencies that the unit under test interacts with. Stubs are typically used when testing code that relies on external systems, such as databases, APIs, or network services, that are either difficult to set up or maintain during testing.
In mocking, we receive fixed data, so adding more products won’t change the result. To address this, we can use stubs, a more realistic form of mocking. With stubs, we create another file called a fakeRepository that implements the repo interface. In this fakeRepository, we define functions like addProduct and getproductCount, keep track of product count using a variable. Each time addProduct is called, we increment the count and return it in the productCount method. This way, if we add one more product after the initial five, the count will correctly reflect as six. We’ll write a simple code to replicate the behavior of the repository. now we will autowire fakeRepository file instead real repo file in ServiceTest.
Here’s an analogy to understand the concepts:
Imagine your mom asks you to go to the temple, and you lie, saying you went, without actually going. This is like mocking.
Then, your mom tells you it will take only 5 minutes, and you return home early. Next time, you go to your friend’s house for 5 minutes and come back. This is more realistic, like a stub.
Now, your mom asks you to go to the temple and her friend’s house, which is on the way to the temple. She calls her friend to confirm, and you go to via her friend’s house and then somewhere else for 5 minutes, and come back lying, saying you went to the temple. This is like faking; it’s more realistic than stubbing, but still not genuine
Fake -> In unit testing, “fake” refers to a simplified implementation of a component or dependency used to replace the real implementation. Fakes are used to simulate the behavior of real components in order to test the system in isolation. Unlike stubs or mocks, fakes are more elaborate and closely mimic the behavior of the real components, providing a more realistic testing environment
There are scenarios where a stub may not suffice. For example, if we want to test the name of an added product, merely increasing the count won’t serve our purpose. To address this, we can use a hashmap data structure to store added products. Instead of saving products in the database, we mimic this by saving them locally. This approach is more realistic than stubbing as it allows us to retrieve the saved product and test its attributes.